Hi Folks! In this post I am going to show how to implement a controller method in ASP.NET Core 6 that resizes images on the fly. First, clone the github repository (it will be much easier to follow this post):
Find the files here.
Open the ResizeImage.sln solution file in Visual Studio. Head straight to a controller called FilesController.cs. You should see something like this:
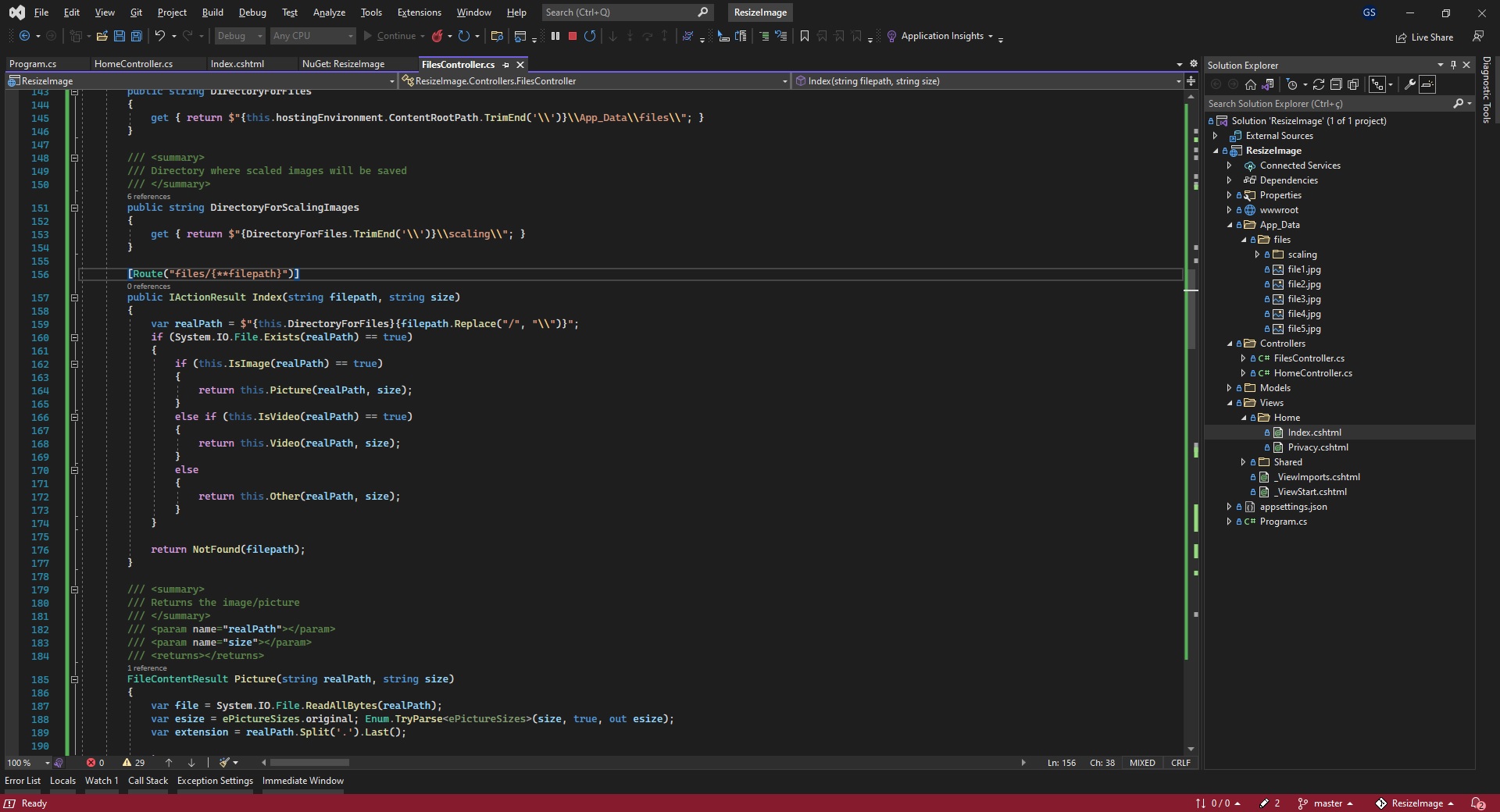
We are going to start with the Index method, identifying which file type the Controller is serving. Only pictures will be resized. The Files controller supports a query parameter called size. This size will be translated to an enum called ePictureSizes and the new width is as follows:
- original (does not scale);
- thumbnail (50px);
- small (200px);
- medium (500px);
- large (1000px).
If the device accessing the website is mobile, the images will be resized to lower widths (take a look at the enum: mobile_thumbnail, mobile_small, mobile_medium and mobile_large).
Now, press F12 on a method called Picture. The most important thing inside this code is, in order to generate resized files with unique names, the full path is used to create a hex string from an md5 hash (lines 218 and 221). If the file already exists in the scaling directory (inside App_Data/files), the file is returned right away. If not, the method ScalePicture is called. Press F12 on it.
The ScalePicure method basically does the dirty work, it calculates the new height and saves the new file. Well, for jpg files, the new files are saved with 50% quality, reducing the file sizes even more. Take a look of how the resized images look like:
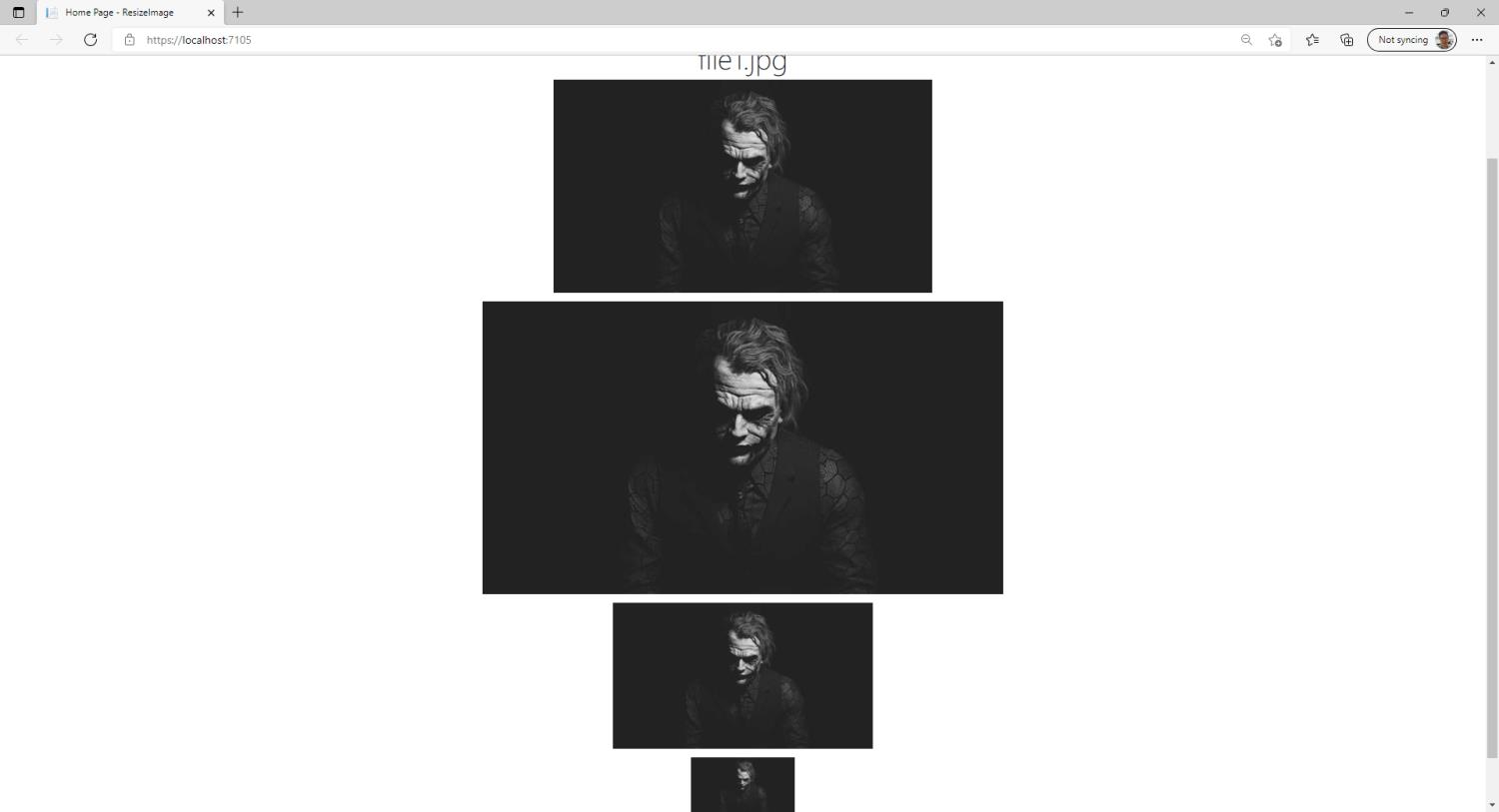
This website only works on Windows, based on the libraries used (and the visual studio recommendation).
I hope you understood the purpose of the solution, helping improve the load times and the performance of websites.
Enjoy!